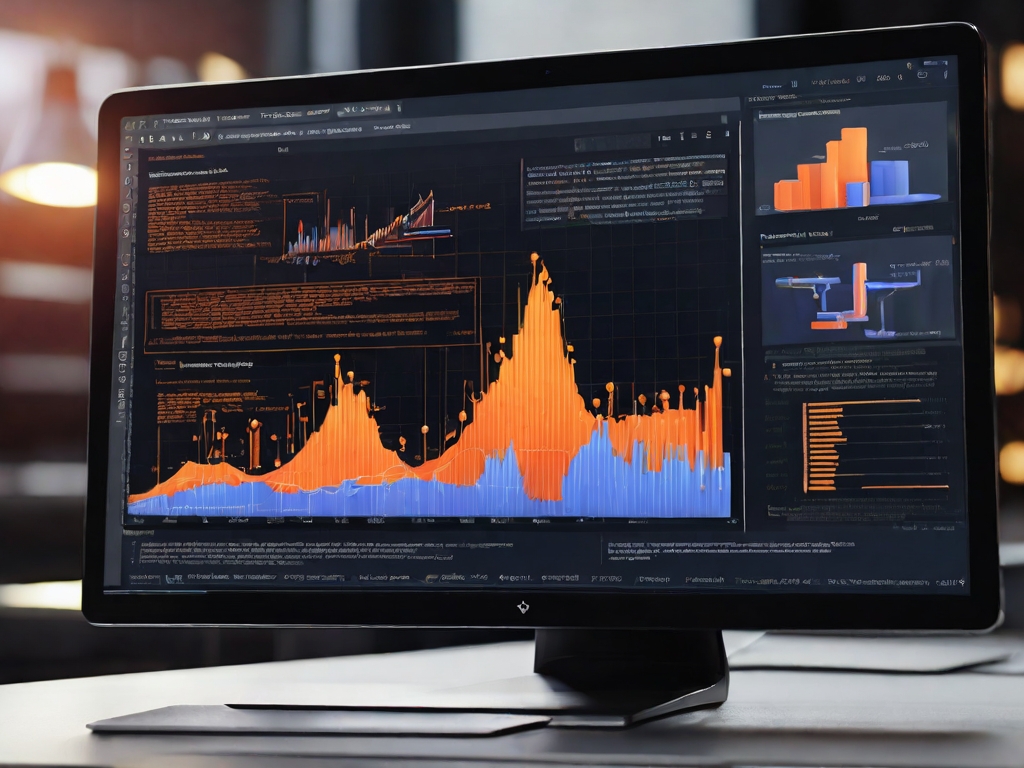
In today’s world, machine learning (ML) and artificial intelligence (AI) are at the forefront of technological innovations. TensorFlow, one of the most popular ML libraries, enables developers to build robust models for a wide range of tasks, from image recognition to health diagnostics. In this article, we will walk through how to scale data and train a TensorFlow model using Python, offering beginners a step-by-step guide to understanding the process.
Why Data Scaling is Crucial for Machine Learning
Data scaling plays a vital role in machine learning because it ensures that your model processes each feature equally. In most datasets, features can vary widely in scale, which can lead to skewed predictions. For instance, if you’re building a health model to predict diabetes, features like “age” may range between 20 and 80, while others like “glucose level” could range between 0 and 300. Without scaling, models may give more importance to the larger numerical features, even if they are not necessarily more important.
One common scaling technique is Min-Max Scaling, which rescales data between 0 and 1. This ensures that each feature is treated equally during training. You can perform scaling easily using Python’s Pandas library, as follows:
df_scaled = (df - df.min()) / (df.max() - df.min())
This approach makes sure that all the numerical features are now between 0 and 1, reducing any bias in the model’s predictions.
Using TensorFlow for Classification Tasks
Once you have scaled your data, the next step is building a model. TensorFlow is an open-source platform specifically designed for creating machine learning models. Let’s break down a simple linear classification model to predict whether someone has diabetes.
Setting Up TensorFlow
Before jumping into the TensorFlow code, you need to import the necessary libraries:
import tensorflow as tf
import pandas as pd
TensorFlow offers an efficient way to handle various feature types, including both numeric and categorical features. To define a numeric feature in TensorFlow, we use the following:
number_pregnancies = tf.feature_column.numeric_column('number_pregnant')
This tells TensorFlow that the column number_pregnant
consists of numeric values ranging from 0 to 1, as it has already been scaled.
Handling Categorical Data
If your dataset includes categorical features such as groups labeled A, B, C, or D, you can define them using TensorFlow’s categorical_column_with_vocabulary_list()
function. This ensures that TensorFlow understands the values as categories and not numerical values.
group = tf.feature_column.categorical_column_with_vocabulary_list(
'group', ['A', 'B', 'C', 'D'])
By defining categorical features this way, TensorFlow can assign values properly without skewing the results by treating categories as numbers.
Visualizing the Data
Another critical step in the data preparation process is understanding your data’s distribution. Using Matplotlib, you can create histograms to visualize how different age groups are represented in your dataset. For example:
codeimport matplotlib.pyplot as plt
df['age'].hist(bins=20)
plt.show()
This shows you how the age feature is distributed across the population, helping you better understand the demographic trends in your dataset.
Training Your TensorFlow Model
Once the data is prepared and visualized, it’s time to train your model. The TensorFlow function model.train()
helps you fit the model to your training data. Here’s a simple setup:
model = tf.estimator.LinearClassifier(feature_columns=feature_columns)
model.train(input_fn=train_input_fn, steps=1000)
In this example, the input_fn
defines how the training data is fed into the model, and the steps=1000
parameter specifies how many times the model iterates over the data during training.
Evaluating and Testing Your Model
After training the model, you can use the test dataset to evaluate its accuracy. This allows you to see how well the model generalizes to unseen data. Using TensorFlow’s model.evaluate()
function, you can measure the model’s performance:
results = model.evaluate(input_fn=eval_input_fn)
The output will give you metrics such as accuracy, precision, and recall. In the example above, the model reached an accuracy of 71%, which is quite promising for a small dataset.
Key Takeaways
By the end of this tutorial, we successfully scaled data, built a TensorFlow model, and evaluated its performance. TensorFlow is a powerful tool for machine learning tasks, and by following these steps, you can start building and testing your own models. As AI continues to evolve, mastering these techniques will provide you with a strong foundation in machine learning.
For further learning, consider exploring advanced topics such as deep learning and neural networks. Remember, machine learning is an iterative process; the more you experiment, the better your models will become!